If
The if statement is the simplest control statement. It checks whether a condition
is true or false. If the condition is satisfied, all the code within the braces '{}' is
executed. The syntax for an if statement is as follows:if (condition)
{
//if true these statements are executed
}The following is an example of an if statement. If the variable a is greater than
10, the value of a will be printed to the screen.if (a > 10)
{
print a;
}The following is an example of an if statement using multiple expressions to
evaluate the condition.if((a < 5) || (a > 10))
{
print a;
}
If...elseAn if statement checks for only one possibility and ignores all other conditions.
An if…else statement checks one condition and if true, the block of code is
executed. Otherwise, an alternative statement is executed. The syntax for an
if…else statement is as follows:if (condition)
{
//if true these statements are executed
}
else
{
//if false these statements are executed
}
int i = 12;
int j = 10;
int max;
if (i > j)
{
max = i;
}
else
{
max = j;
}The previous conditional formulas allow for only two alternative outcomes. A
program might have to check more than two alternatives. To check for multiple
alternatives, you can use an if…else...if statement. The syntax for this statement
is as follows:if (condition1)
{
//statement1
}
else
{
if (condition2)
{
//statement2
}
else
{
//statement3
}
}
The system checks condition 1 first, and if satisfied, statement1 is executed. If
the condition 1 is not satisfied, it moves to condition2. If condition2 is satisfied,
statement2 is executed. If condition2 is not satisfied, then statement3 executes.
You can use as many conditions as necessary.
You might need to have a condition in a condition. You can do this using nested
if statements.
A mathematics teacher uses the following criteria to determine who passes or
fails the class:• Pass the final exam• Pass the homework sections
Check the logic in the following order:
1. If the student has failed the exam, then the student fails the course.
2. If the student has passed the exam, then check the student's
homework sections.
3. If the student has passed the exam and the homework sections, the
student passes the course.
4. Otherwise, the student fails.boolean passExam = true;
boolean passHomeWork = false;
str studentStatus;
if (passExam == true)
{
if (passHomeWork == true)
{
studentStatus = "Passed";
}
else
{
studentStatus = "Failed";
}
}
else
{
studentStatus = "Failed";
}
Best Regards,
Hossein Karimi
Friday, June 29, 2018
If Statement
Labels:
X++
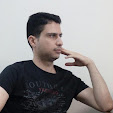
Subscribe to:
Post Comments (Atom)
Configure the Firewall on the Enterprise Portal Server
After you install Enterprise Portal, enable Web Server (HTTP) in Windows Firewall. If you do not enable the web server in Windows Firewall...
-
To integrate Microsoft Dynamics AX and Analysis Services, you must connect Analysis Services to the Application Object Server (AOS). To do s...
-
The below select query will give the both the Sales Line record count and the sum of sales quantity. static void Test_Data(Args _args) ...
-
A List object contains members that are accessed sequentially. Lists are structures that can contain members of any X++ type. All the member...
No comments:
Post a Comment