A switch statement acts as a multi-branch control statement that defines an
expression and whose result leads to a specific program execution. The switchstatement considers the result and executes code, depending on possible
outcomes of the expression. These are known as cases. Each of these cases is
listed in the body of the statement.
Following the colon after each case are statements that execute if the expression
satisfies the case. There can be any number of statements following a case in aswitch statement. The body of a switch statement is enclosed in braces '{}'. The
following shows the syntax for a switch statement:
switch (expression)
{
case 'Choice1':
Statement1;
Statement2;
break;
case 'Choice2':
Statement3;
break;
case 'Choice3':
Statement4;
Statement5;
Statement6;
break;
default : DefaultStatement;
}
The break; statement tells the program to leave the switch statement and
continue immediately after the switch. This can also be used elsewhere in X++
coding. The default case executes if the result of the expression does not match
any of the cases. Using the default case is optional.
The following example compares a switch statement with an if…else statement.
For these examples, students receive a score from a test. The score must have a
corresponding letter grade. The scores can only be 90, 80, 70, and so on.int score = 80;
str grade;
str message;
switch (score)
{
case 90 : grade = "A";
message = "Excellent";
break;
case 80 : grade = "B";
message = "Good";
break;
case 70 : grade = "C";
message = "Average";
break;
case 60 : grade = "D";
message = "Poor";
break;
default : grade = "Failed!";
message = "You need to study more!" ;
}This example produces the same result as the previous example, but uses if...elsestatements instead of the case statement. Note the number of lines of code and
the ease of reading the code in each case.int score = 80;
str grade;
if (score == 90)
{
grade = "A";
}
else
{
if (score == 80)
{
grade = "B";
}
else
{
if (score == 70)
{
grade = "C";
}
else
{
if (score == 60)
{
grade = "D";
}
else
{
grade = "Failed!";
}
}
}
}As illustrated on the previous page, the switch statement simplifies the code and
makes the program flow more efficiently. Another advantage of using a switchstatement is allocating multiple results of the expression to one outcome or case.
The following example shows the use of multiple expressions in a switchstatement.str color = "red";
str colortype;
switch (color)
{
case "red", "yellow", "blue" :
colortype = "Primary Color";
break;
case "purple", "green", "orange" :
colortype = "Secondary Color";
break;
default : colortype ="Neither Primary or Secondary";
}
Best Regards,
Hossein Karimi
Friday, June 29, 2018
Switch Statement
Labels:
X++
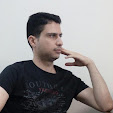
Subscribe to:
Post Comments (Atom)
Configure the Firewall on the Enterprise Portal Server
After you install Enterprise Portal, enable Web Server (HTTP) in Windows Firewall. If you do not enable the web server in Windows Firewall...
-
The below select query will give the both the Sales Line record count and the sum of sales quantity. static void Test_Data(Args _args) ...
-
In Reporting Services, reports and resources are processed under the security identity of the user who is running the report. If the report ...
-
A List object contains members that are accessed sequentially. Lists are structures that can contain members of any X++ type. All the member...
No comments:
Post a Comment